The setup
- Data file
- We will using a local json file to act as our data source
- The data is our sample contact information we have been using for some time.
- var data_file: string = ‘assets/data.json’;
- We will update the name of the file so that the httpclient will not find it
- Load the file via Http get request
- We will use Angular’s built in Httpclient to make the request
- In each case we will set the number of times or when we want to retry
- The case of when is a more advanced case but if you follow this example it will be pretty easy to use in your own internal application
Create a new UI component
Ng g c views\contact – contacts.component.ts
- Add the method calls
- RetryWhen() –
- W will use this to perform retires with a timed delay when there is an error.
- Retry3() –
- This performs our mandatory 3 additional tries after our first attempt, by angular flow the operations a performed before your notified of the final error.
- FetchData()
- This fetches all the data from the service the takes only 3 items from the set, this demonstrates one other nice feature which is filtering on the client site, you may not need this one , but I found many tutorials did not outline how you would perform this operation when returning all your data on one go.
- RetryWhen() –
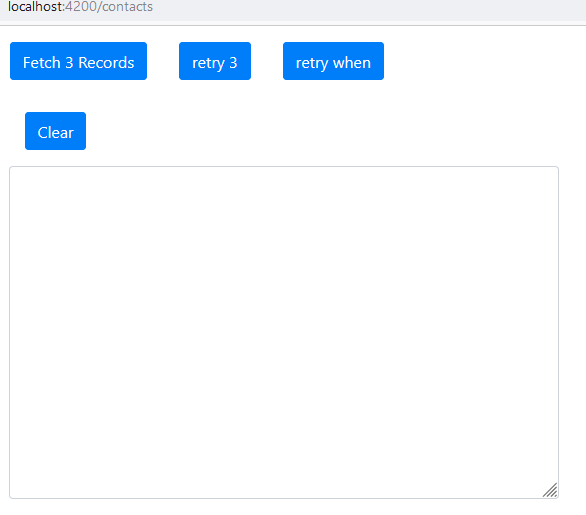
public RetryWhen()
{
this.retryWhensubscrip = this.timeService.contactSubject
.subscribe((result: Contact) =>
{
this.Contacts.push(result as Contact);
});
this.timeService.RetryWhen();
}
/** */
public Retry3()
{
this.Retry3Subscrip = this.timeService.contactSubject
.subscribe((result: Contact) =>
{
this.Contacts.push(result as Contact);
}, (err) => { }, () =>
{
});
this.timeService.Retry3();
}
/** */
public FetchData()
{
let subscrip = this.timeService.contactSubject.
pipe(take(3))
.subscribe(result =>
{
this.Contacts.push(result as Contact);
}, (err) => { }, () =>
{
subscrip.unsubscribe();
});
this.timeService.FetchTheDataAll();
}
Other methods neeed to prevent memory bugs and cleanup
/** */
Clear() { this.message = ''; }
ngOnDestroy(): void
{
this.uiNotifysubscrip?.unsubscribe();
this.retryWhensubscrip?.unsubscribe();
this.Retry3Subscrip?.unsubscribe();
this.FetchDataSubscrip?.unsubscribe();
}
ngOnInit(): void
{
/**/
this.uiNotifysubscrip = this.timeService?.uiNofify
.subscribe((data) =>
{
this.message += `${data}\n\r`;
});
}
Create the service
Add the methods
- FetchTheDataAll()
- Retry3(ntimes: number = 3)
- RetryWhen()
/** */
public FetchTheDataAll()
{
let subscrip = this.http.get<Contact[]>(this.data_file)
.pipe(catchError(
(error: HttpErrorResponse) =>
{
let merror = error.message;
this.uiNofify.emit(merror);
return of(merror);
}))
.subscribe((result) =>
{
if (result! instanceof String) {
let resultTemp = result as Contact[];
resultTemp.forEach(item => this.contactSubject.next(item));
}
subscrip.unsubscribe();
});
}
/**
*
* @param ntimes
*/
public Retry3(ntimes: number = 3)
{
let subscrip = this.http.get<Contact[]>(this.data_file, {})
.pipe(retry(ntimes)
, catchError(
(error: HttpErrorResponse) =>
{
let merror = error.message;
this.uiNofify.emit(`All attempts failed! \r\n${merror}`);
return of(merror);
})
)
.subscribe((result: Contact[] | string) =>
{
if (result! instanceof String) {
let resultTemp = result as Contact[];
resultTemp.forEach(item => this.contactSubject.next(item));
}
subscrip.unsubscribe();
}, (error) => { });
}
public RetryWhen()
{
let subscrip = this.http.get<Contact[]>(this.data_file)
.pipe(retryWhen(error =>
error.pipe(
scan((acc, error) =>
{
if (acc > 3) throw error;
this.uiNofify.emit(("attempt " + acc));
return acc + 1;
}, 1)
, tap(val =>
{
})
, delayWhen(val => timer(5 * 1000))))
)
.subscribe((result) =>
{
let resultTemp = result as Contact[];
resultTemp.forEach(item => this.contactSubject.next(item));
subscrip.unsubscribe();
});
}
RetryWhen
The timer allows a delart of 5 seconds before we try again,
this can be set to days if needed
The audio was bad enough for me to remove it
You can find the sourece at my gihub account https://github.com/caribtechnet/auto_retry_angular_http_request